Creating interactive web pages is one of the most exciting things you can do as a web developer. JavaScript, often referred to as the “language of the web,” allows you to make web pages dynamic and user-friendly. If you’re just getting started or want to enhance your existing skills, this guide will show you how to use JavaScript for interactive web pages in simple terms, with clear examples.
What is JavaScript, and Why is it Important?
JavaScript is a programming language used primarily to make web pages interactive. While HTML provides the structure of a web page and CSS handles the design, JavaScript adds life to your website by enabling users to interact with elements such as buttons, forms, images, and much more.
With JavaScript, you can:
- Add interactive forms
- Create pop-ups and alerts
- Dynamically change the content of the page
- Respond to user actions like clicks and key presses
Let’s dive into the world of JavaScript and see how it works with a few simple and practical examples.
How to Use JavaScript for Interactive Web Pages
Basic Setup: Adding JavaScript to Your Web Page
Before you start writing JavaScript, you need to include it in your HTML file. There are two main ways to do this:
Inline JavaScript: You can write JavaScript directly inside your HTML file using the <script> tag.
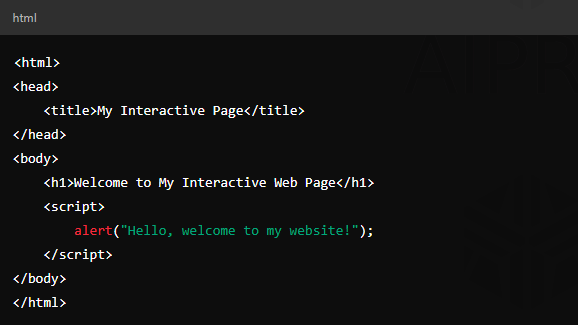
- In this example, a pop-up alert will show up when someone opens the page. It’s the simplest way to include JavaScript.
External JavaScript: Another option is to link to an external .js file.
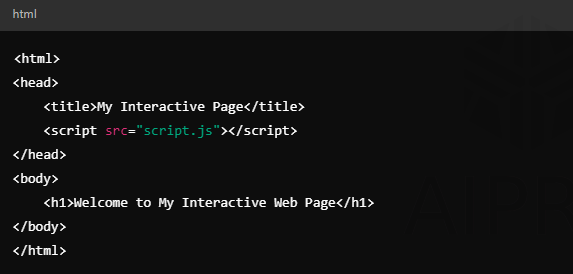
- This is a cleaner approach, especially for larger projects. The JavaScript code will be placed in a separate file named script.js.
How to Make Your Web Page Interactive with JavaScript
Responding to User Actions with Event Listeners
An event listener allows JavaScript to “listen” for user actions, such as clicks, key presses, or mouse movements. Here’s an example of using a button and an event listener to trigger an action when the button is clicked.
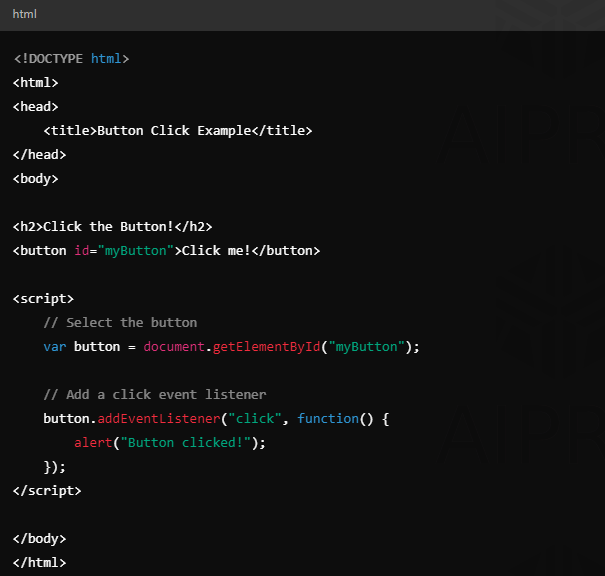
In this example, when the user clicks the button, a pop-up will appear with the message “Button clicked!” This is how JavaScript responds to user input.
Manipulating HTML Elements with JavaScript
JavaScript can dynamically change the content of a web page. For example, you can modify text or images when a user interacts with your page.
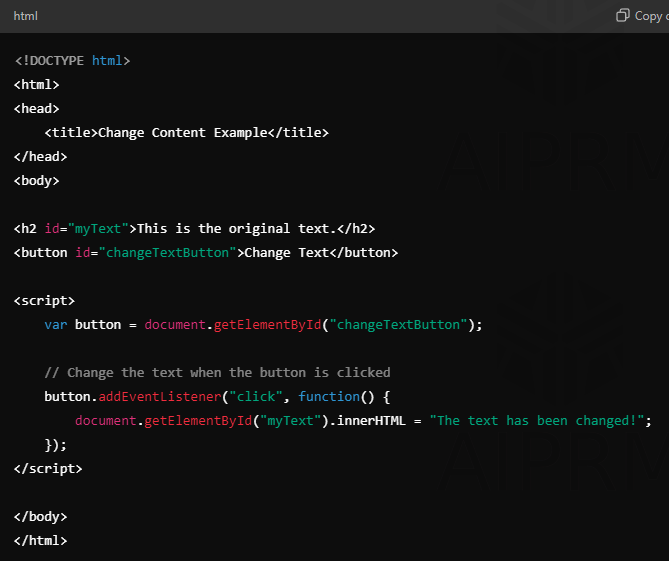
In this example, the text inside the <h2> tag will change when the button is clicked. This is a simple but powerful way to make your page more interactive.
Validating Forms Using JavaScript
One of the most practical uses of JavaScript is validating forms. Before a form is submitted, JavaScript can check if the input values are correct. Here’s an example of a basic form validation:
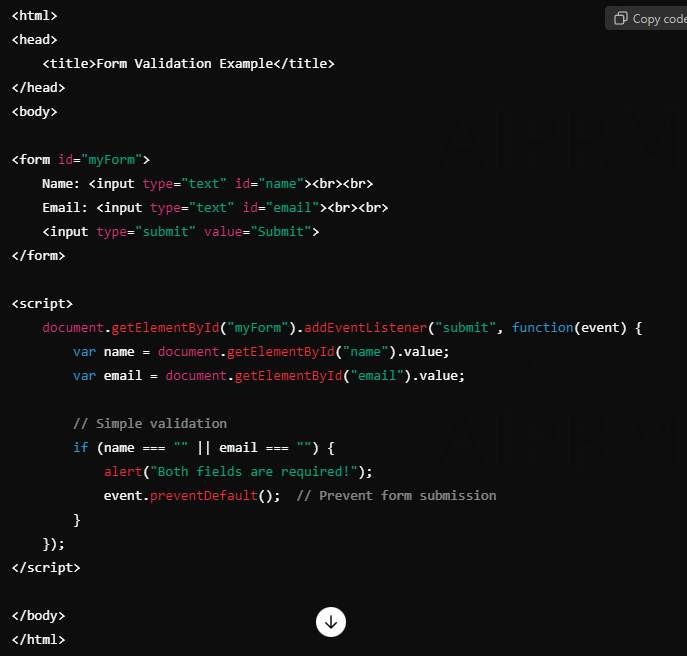
Here, JavaScript checks whether the user has filled in both the name and email fields before submitting the form. If either field is empty, an alert will pop up, and the form won’t be submitted until the fields are completed.
Changing Styles with JavaScript
JavaScript can also change the CSS of an element dynamically. You can modify colors, font sizes, and other styles when a user interacts with the page. Here’s an example of changing the background color when a button is clicked:
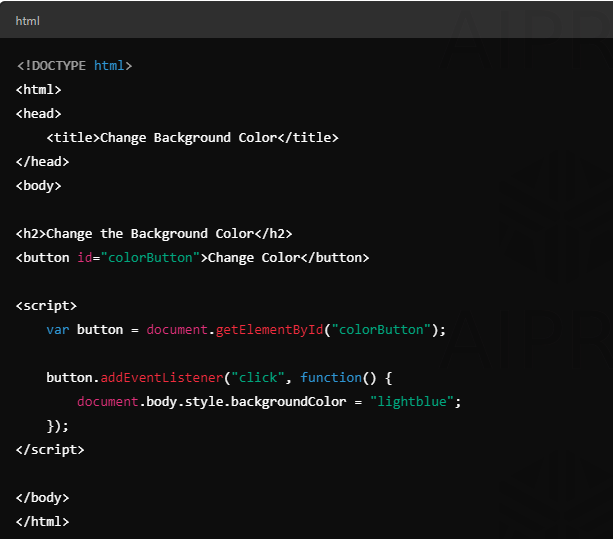
In this example, when the user clicks the button, the background color of the page changes to light blue. You can use this to create dynamic, visually engaging effects on your website.
Interactive Examples: Tables and Lists with JavaScript
JavaScript is great for manipulating tables and lists dynamically. Let’s explore how to create interactive tables and lists.
Dynamically Adding Rows to a Table
JavaScript makes it easy to let users add rows to a table dynamically.
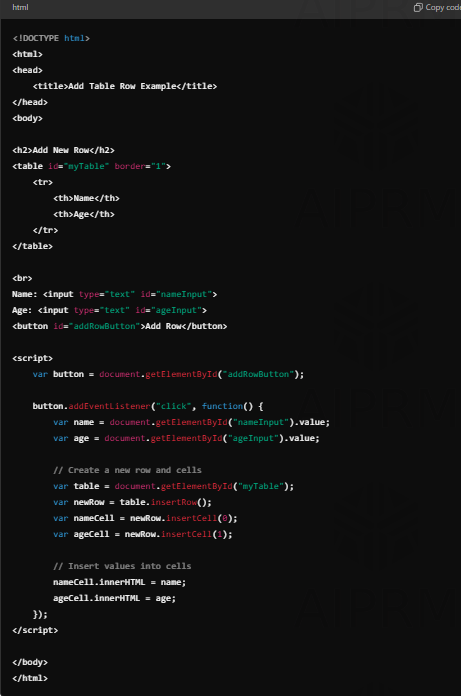
This example allows the user to enter a name and age, and a new row with that data will be added to the table every time the button is clicked.
Dynamically Adding Items to a List
Similarly, you can let users add items to a list dynamically:
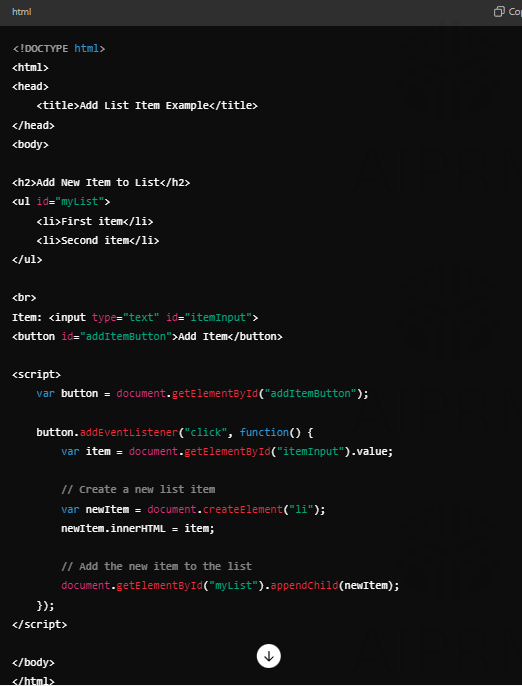
With this example, users can add new items to a list by entering text into the input field and clicking the button.
Also Read: Top 30 Javascript Project Ideas For Portfolio With Source Code
Conclusion
JavaScript is a powerful and versatile language that can make your web pages interactive and engaging. From responding to user actions, changing content dynamically, validating forms, and modifying styles to adding new elements to your page, the possibilities are endless.
By understanding the basics of JavaScript and how it interacts with HTML and CSS, you can create user-friendly and dynamic web experiences that keep visitors engaged.
Remember, practice is key. Try the examples above, experiment with your own code, and continue learning. As you grow more comfortable with JavaScript, you’ll be able to add more advanced interactivity to your web pages.