Java is a high-level language and an object-oriented programming language. OOP is a paradigm that focuses on objects, which are collections of data called attributes, and functions or procedures known as methods. This is different from a procedural program, where one is mainly concerned with procedures or functions that work on data. In this blog, you will read the key Difference b/w Class and an Object in Java with real-world examples.
The core principles of OOP are:
Encapsulation: involves packaging the data on which the methods work and the methods into one unit or class.
Abstraction: The concept of concealing the internal workings of a system and presenting only what it is capable of doing.
Inheritance: The process by which one class derives properties or behavior from another class that has already defined them.
Polymorphism: By definition, this makes an operation polymorphic—methods can have the same name but behave differently depending on what they are operating over.
It is now apparent that every object-oriented programming language, including Java, was developed to employ all these concepts in modeling real-life objects and systems.
What is a Class in Java?
In Java, a class can be described as a straightforward blueprint or form that outlines how objects should be created. It explains the shape and characteristics of the objects to be produced from it, including data and methods. A class is not a storage of any data; it simply tells you how objects can be created.
In fact, a class is like a blueprint for a house. The blueprint (class) outlines the number of rooms and arrangements, but it is made of something other than bricks. It is only when the house (object) has been constructed that it gains characterization in terms of materiality.
Components of a Class
A class in Java typically consists of:
Fields/Attributes: These contain the state or data of the object as it is referred to in-class lessons.
Methods: These are the activities that indicate operation in the object.
Constructors: These are methods that are invoked at the time an object is created from the class.
Access Modifiers: Terms such as public, private, and protected that characterize the state of accessibility of the class and its constituents.
Syntax of a Class
public class Car {
// Attributes (fields)
String brand;
String model;
int year;
// Constructor
public Car(String brand, String model, int year) {
this.brand = brand;
this.model = model;
this.year = year;
}
// Method (behavior)
public void StartEngine() {
System.out.println(“The engine of ” + brand + ” ” + model + ” is started.”);
}
}
In this example, the class Car has three fields (brand, model, year), and method startEngine (). This also contains a constructor that sets the values of attributes of the object being formed at the time of the creation of the object of the Car.
3. What is an Object in Java?
An object is a practical example of a class. It is the real thing that possesses information and can execute activities associated with the techniques described in the class of the object. Objects are usually called instances because they are concrete examples of a class. An Object is an entity having a state, which is exhibited by fields or attributes, and behavior, which is exhibited by methods.
To put it more simply, while a class is a design, an object is actually a structure built from that design plan.
Creating an Object
In Java, to instantiate an object, you type new before the class name; this allocates space for an object of that particular class and returns a reference to the object.
Example:
Car myCar = new Car(“Toyota”, “Corolla”, 2020);
myCar.StartEngine();
In this case, myCar is an instance of Car. The new Car class construction(“model,” “model,” year): “Toyota,” “Corolla,” 2020 creates a new object of the class Car.
Object Characteristics
State
Attributes are used to describe the Probability of the object in question at a given time if it has to be challenged. For instance, in my state, the brand is “Toyota, the model is “Corolla, and the year is 2020.
Behavior
That is why the ‘StartEngine’ method can be regarded as a behavior in an object that can be initiated. When this method is called, it turns on the car’s engine, changing its state from state_engine_off to state_engine_on.
Identity
Every object has its identifier, which can be the same as that of some other objects, although its state can also be the same as that of other objects.
Difference b/w Class and an Object in Java
Knowledge of what the class is and what an object is remains essential when working with Java language. Below are the key distinctions:
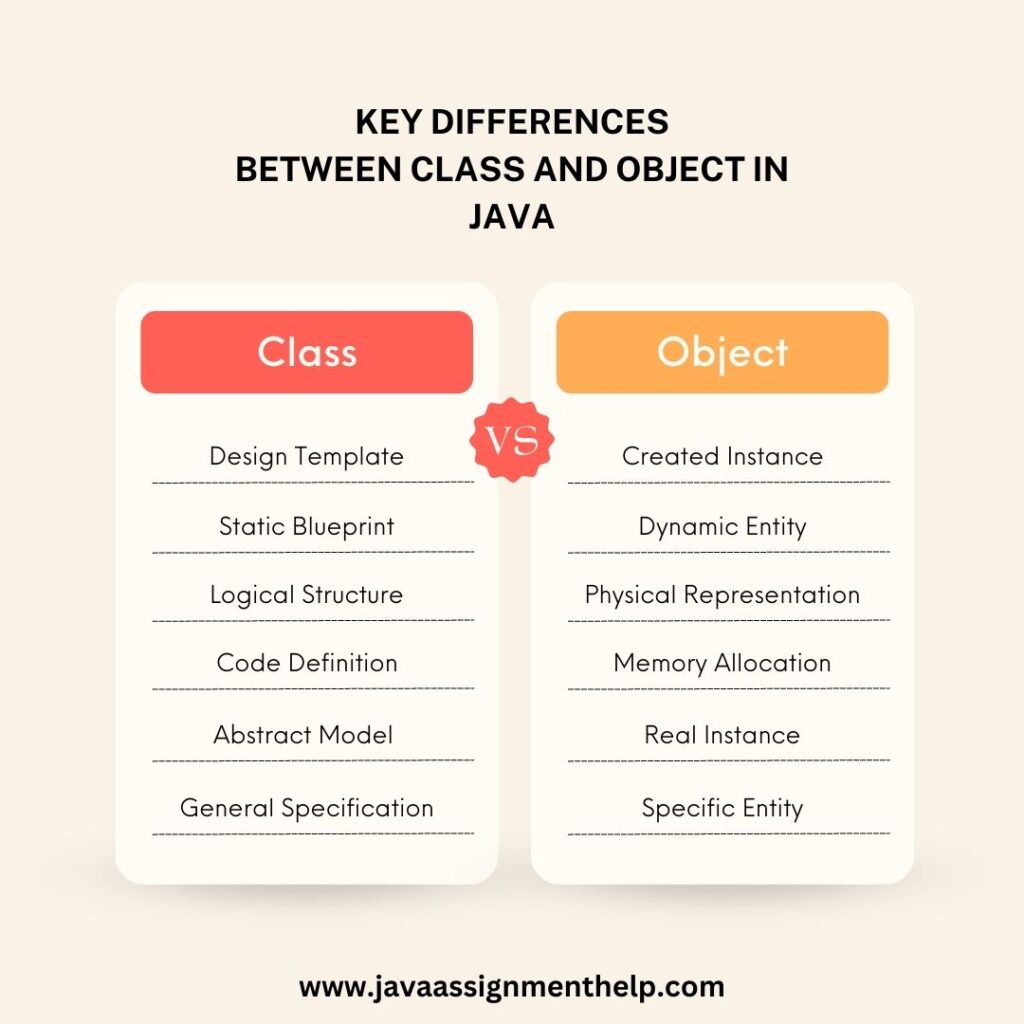
1. Blueprint vs. Instance
Class: A class is a form with the structural and methodological features of objects. But it has no real or recorded information.
Object: An object is an instance of a class. It provides real information and is involved in notification with other parties in some programs.
2. Memory Allocation
Class: In fact, no memory space is created when a class is declared. The class is implemented solely in the source code to help instantiate objects.
Object: Memory is acquired when objects are created using new keywords. Depending on their size, memory can increase or decrease. Every object is a point in memory; it has its data (state).
3. Data Representation
Class: Unlike a class that does not contain data, a class defines how data will look within objects.
Object: An object encapsulates real data and the procedures to process this data. For instance, the Car class doesn’t contain any specific car data until you create objects such as the myCar object and the yourCar object.
4. Behavior and Actions
Class: The class defines the set of actions that an object may use, but the class itself does not act.
Object: Objects can also perform actions such as starting an engine or calculating a value by simply calling the class’s methods.
5. Reusability
Class: Classes, in this case, are like blueprints. You can have many individual objects of a particular class, yet the structure and behaviors of that class do not need to be created new.
Object:
Each particular object stores data to which it alone has access, while all objects possess the same attribute structure if they exist in the same class.
The Role of Constructors
A constructor is an instance of a class that is used whenever an object is to be created. It is mainly used to set the first value of the object’s properties at the time when the object is created.
Default Constructor: Unlike in C++, if you do not explicitly specify the constructor of your class, Java enables a default constructor, which initializes the object’s attributes to default values: null for objects and 0 for the numeric types.
Parameterized Constructor: This is also a good place to call a parameterized constructor to set the initial values of attributes, as shown above.
Also Read: 29+ Exciting Java Project Ideas for All Skill Levels
Concepts Related to Classes and Objects
1. Static vs. Non-Static Members
Static Members:
Approximers are class fields or methods and the latter is not an object in some object. That is, as some would have it, static members of a class are its properties and the usage of the member in question is applied to all instances of the class.
Non-Static Members: Each class object has at least one non-static field or method. These members are depicted here as images of a single production object.
Example:
public class Car {
static String manufacturer = “General Motors”; static field
It contains string model; // Non-static field
public void display() {
console.writeline(manufacturer + “ makes “ + model)
}
}
2. The Object Class
In Java, each class has an Object class as its immediate superclass, regardless of whether it is declared in the class header. The Virtual Object type in Java has many important methods. For all objects of Java, it is possible to get to, for example, toString(), hashCode() and equals().
3. Garbage Collection and Object Lifecycle
Java also employs automatic memory management through garbage collecting, where the JVM collects memory used by objects that are no longer in use. Some Java programs come with garbage collection, but knowing when objects are collectible requires a significant effort in optimizing the resources used.
Relationship between Class and Objects?
The relationship between a class and an object is essential in OOP:
Class to Object: A class is said to be a blueprint for making objects. It prescribes the characteristics and the process that an object is to exhibit.
Object to Class: Objects are the particular example of the class. They are the runtime entities that hold data to perform operations and are involved in operations between them.
In short, classes are only the declaration of a set of objects, and objects are actual instances of objects created during the runtime.
Conclusion
It seems there is no better way of introducing the much-hyped object-oriented programming in the context of Java than to start by explaining the crucial difference between two basics that every programmer should be wholly aware of the class and the object. While they are very similar, they serve different purposes and are located at different levels of the application architecture.
A class can be seen as a blueprint, a template or even a design specification for how objects need to be built and which actions they are capable of. It enables the definition of attributes (fields) and behaviors (methods) while not being able to store information by itself. The class mainly resides at the level of concept definition in the code.
Therefore, classes act as templates that give an application structure and form, and objects are the actual, tangible, real-world manifestations of those forms. Together, they constitute the heart of Java’s object-oriented organizational.
FAQs
What is the biggest distinction between a class and an object in Java?
A class is a blueprint of objects and the methods associated with them in addition to a set of values that virtually defines what the object should be However, an object as known as an instance is the actual version of this class in form of data and actions.
When is memory allocated for a class and an object?
Array does not allocate memory for just a class but it does allocate memory for the objects created from that class. Actually objects occupy memory since they contain real data while the class just offers an outline.